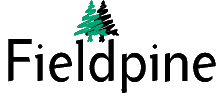
EventSource Realtime data feed
Javascript has a widely available but not well known feature called EventSource() or 'Server Sent Events' (SSE). You can read more about these here. The file WebUi.js opens an event source connection and uses these updates to generate the onMessage events that are sent to your javascript.
If you are not using WebUi.js and are directly interfacing then you probably want to create an EventSource() to receive updates. However there are maximum connection limitations around using eventsource, so you should open these sparingly. If you've never used EventSource before there are some samples at the end of this page using both EventSource() and fetch()
Requesting an Event Source
The following query arguments are available
Name | Purpose |
format | Event Format. Requests event stream data to be delivered in an alternative format. The default format is JSON |
want | A comma seperated list of event data you wish to receive. The default value is "sale" which indicates the event source is being used to runnnig or monitoring active sales |
session | Required. Supplies the session identifier for where events are to be sourced from. |
Contents of the Source
All event type names are in lowercase unless specifically documneted. There are some mixed case event types which are used for rare or more diagnostic type messages
sale
usermessage
userprompt
barcode
Field | Purpose |
RawScan | The raw barcode scan data |
Owner |
Indicates the owning session for this scan. Scan advisories are sent to all subscribers and this field indicates if you should process this message or not
0 - You are receiving this message as an advisory and can ignore it 1 - This message is for you to display and process 2 - This message is being sent to multiple subscribers. It isn't clear to the Pos Engine which session should receive this advice |
TypeCode | A numeric value indicating the type of barcode this appears to be |
TypeStr | A string value equivalent of TypeCode |
The Last-Event-Id is set to the RVE of the records change date/time in UTC. This means it will be a value in the format YYYYMMDD.HHmmSSCCC
Using EventSource from Javascript
EventSource themselves are incredibly easy to work with, simply open a URL and wait for events to be dispatched to you. The downside is that you cannot add extra HTTP headers or make other adjustments.
// Create the event source var evtsrc = new EventSource("/eventsource?session=????"); // Create a handler for sale // This is triggered whenever a sale update is sent from the server evtsrc.onsale = function (oo) { // oo is a MessageEvent if ((oo.data.length > 4) && (oo.data[0] === '{')) { try { // Your code here.... } catch (_) { ; } } };
If you need to add HTTP headers, then you can use fetch streams instead